Creating a login page or signup page in HTML and CSS is an essential skill for web developers. A well-designed login form ensures a user-friendly experience and is a core feature of websites requiring user authentication. In this blog, you’ll learn how to build a login page and signup page from scratch, with sample HTML and CSS code. This tutorial covers every detail you need to create a login form in HTML and signup form with CSS.
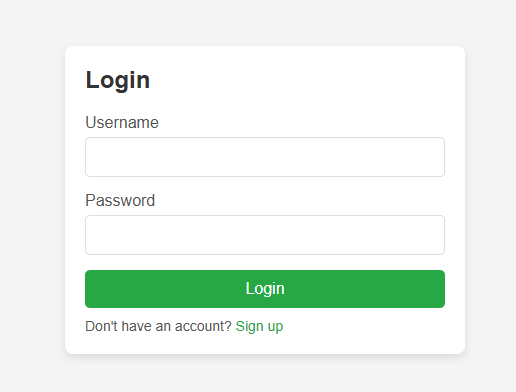
What You Will Learn
- How to set up a simple HTML structure for a login page.
- How to style the login form and signup form with CSS.
- Code examples to make the page responsive and visually appealing.
Step 1: Setting Up the HTML Structure for login Page
To start, create an HTML file called index.html
. This file will contain the structure for both login and signup forms. The example below demonstrates how to use HTML to define the login page layout, including input fields for username and password.
HTML Code for Login and Signup Page
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Login and Signup Form</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="container">
<!-- Login Form -->
<div class="login-form">
<h2>Login</h2>
<form action="#">
<label for="username">Username</label>
<input type="text" id="username" name="username" required>
<label for="password">Password</label>
<input type="password" id="password" name="password" required>
<button type="submit">Login</button>
<p>Don't have an account? <a href="#" onclick="showSignup()">Sign up</a></p>
</form>
</div>
<!-- Signup Form -->
<div class="signup-form" id="signup-form">
<h2>Sign Up</h2>
<form action="#">
<label for="new-username">Username</label>
<input type="text" id="new-username" name="new-username" required>
<label for="new-email">Email</label>
<input type="email" id="new-email" name="new-email" required>
<label for="new-password">Password</label>
<input type="password" id="new-password" name="new-password" required>
<button type="submit">Sign Up</button>
<p>Already have an account? <a href="#" onclick="showLogin()">Login</a></p>
</form>
</div>
</div>
<script>
function showSignup() {
document.querySelector('.login-form').style.display = 'none';
document.querySelector('#signup-form').style.display = 'block';
}
function showLogin() {
document.querySelector('.login-form').style.display = 'block';
document.querySelector('#signup-form').style.display = 'none';
}
</script>
</body>
</html>
Explanation of HTML Code
- Container: The
<div class="container">
is used to contain both the login form and signup form. - Login Form: A basic login page with fields for username and password, and a button to submit.
- Signup Form: A form with fields for username, email, and password.
- JavaScript Toggle: The JavaScript functions
showSignup()
andshowLogin()
toggle between the login page and signup page.
Step 2: Adding CSS Styling
Now that the HTML structure is ready, let’s add CSS to style the login page. Create a CSS file called style.css
and add the following code.
CSS Code for Login and Signup Page
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: Arial, sans-serif;
}
body {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: #f4f4f4;
}
.container {
width: 100%;
max-width: 400px;
padding: 20px;
background-color: #ffffff;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
border-radius: 8px;
}
h2 {
margin-bottom: 20px;
color: #333;
}
form {
display: flex;
flex-direction: column;
}
label {
margin-bottom: 5px;
color: #555;
}
input {
margin-bottom: 15px;
padding: 10px;
border: 1px solid #ddd;
border-radius: 5px;
font-size: 16px;
}
button {
padding: 10px;
background-color: #007bff;
color: white;
border: none;
border-radius: 5px;
font-size: 16px;
cursor: pointer;
}
button:hover {
background-color: #0056b3;
}
p {
margin-top: 10px;
font-size: 14px;
color: #555;
}
a {
color: #007bff;
text-decoration: none;
}
a:hover {
text-decoration: underline;
}
.signup-form {
display: none; /* Hides the signup form initially */
}
Explanation of CSS Code
- General Styles: We set a basic style for the body, headings, and container. The container centres the form on the screen.
- Input Fields: Styled with padding, border-radius, and font-size for a clean look.
- Button: A button with a background color and hover effect, improving the user experience.
- Toggle Display: The
.signup-form
is hidden by default usingdisplay: none;
. JavaScript then toggles between forms.
Step 3: Testing and Finalizing
Open the index.html
file in your browser. You should see a login page with an option to switch to the signup form. When you click “Sign up,” the signup page will appear, and clicking “Login” will toggle back to the login page.
Additional Tips
- Responsive Design: The design is already responsive due to the
max-width
and centering, so it will look good on both mobile and desktop devices. - Customizations: Feel free to change colors, fonts, and button styles in the CSS to match your website’s theme.
- Security Note: This HTML login page does not include backend security, so make sure to implement appropriate security measures for real-world projects.
Conclusion
Creating a login page and signup form using HTML and CSS is straightforward and gives you control over the design and layout. By following this tutorial, you’ve built a functional and responsive login and signup page that users will find visually appealing and easy to use. A well-crafted login page enhances user experience, contributing positively to your website’s credibility.
With this guide, you’re ready to create a stylish login form in HTML. Whether it’s for a personal project, blog, or business website, having a login page and signup page is essential for user interaction.