How to Make a Registration Form in HTML CSS Code:
Build a responsive signup and registration form using free HTML CSS code. Learn easy steps for creating mobile-friendly forms with perfect alignment.
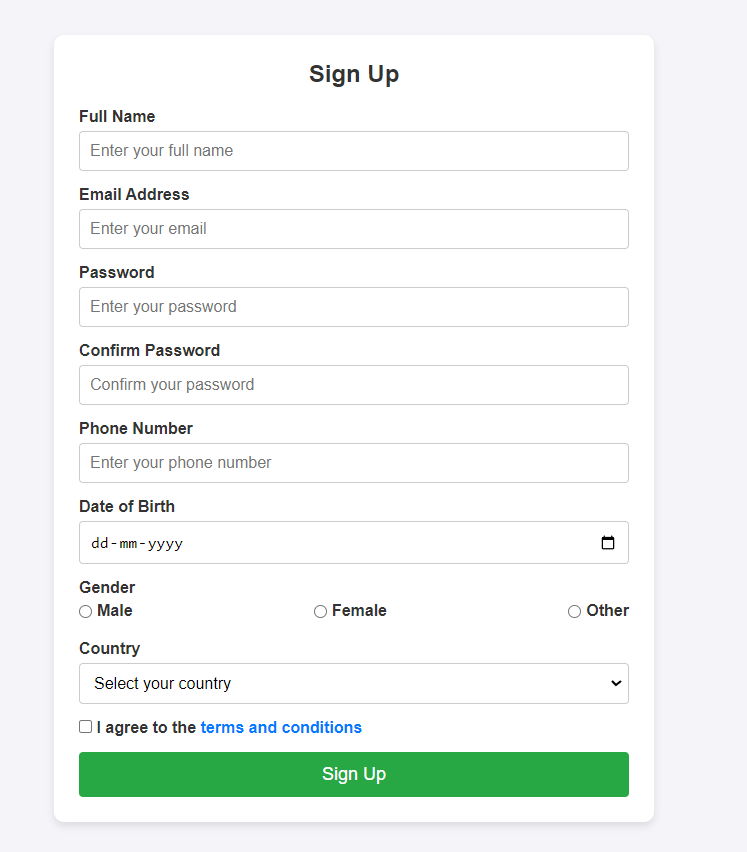
We will also cover the following key points:
- Signup form in HTML CSS
- Adding fields for users to input their information
- How to properly style and align the form
- Best practices for creating a clean and responsive form
- Step-by-step explanations to help you follow along easily
This blog is perfect for beginners or students who are learning how to use HTML CSS code to build forms. Let’s get started!
What is a Signup Form?
A signup form is a simple form that allows users to create an account or register on a website. The form usually contains fields such as:
- Full Name
- Email Address
- Password
- Confirm Password
- Other optional information like phone number, address, etc.
Signup forms are essential for websites where user interaction is required. Now, let’s see how we can create a signup form using HTML CSS code.
Step 1: Structuring the Signup Form with HTML
First, we’ll create the basic structure of the form using HTML. HTML is responsible for laying out the content and the different fields in your signup form. You can create a form template in HTML CSS code that includes input fields for the user’s information.
COPY THIS CODE SNIPPET:
<!-- HTML code snippet -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Responsive Signup Form</title>
<link rel="stylesheet" href="style.css"> <!-- Linking to external CSS file -->
</head>
<body>
<div class="signup-container">
<form class="signup-form">
<h2>Sign Up</h2>
<!-- Full Name Input -->
<div class="form-group">
<label for="fullname">Full Name</label>
<input type="text" id="fullname" name="fullname" placeholder="Enter your full name" required>
</div>
<!-- Email Input -->
<div class="form-group">
<label for="email">Email Address</label>
<input type="email" id="email" name="email" placeholder="Enter your email" required>
</div>
<!-- Password Input -->
<div class="form-group">
<label for="password">Password</label>
<input type="password" id="password" name="password" placeholder="Enter your password" required>
</div>
<!-- Confirm Password Input -->
<div class="form-group">
<label for="confirm-password">Confirm Password</label>
<input type="password" id="confirm-password" name="confirm-password" placeholder="Confirm your password" required>
</div>
<!-- Phone Number Input -->
<div class="form-group">
<label for="phone">Phone Number</label>
<input type="tel" id="phone" name="phone" placeholder="Enter your phone number" required>
</div>
<!-- Date of Birth Input -->
<div class="form-group">
<label for="dob">Date of Birth</label>
<input type="date" id="dob" name="dob" required>
</div>
<!-- Gender Selection (Radio Buttons) -->
<div class="form-group">
<label>Gender</label>
<div class="gender-options">
<label for="male">
<input type="radio" id="male" name="gender" value="male" required> Male
</label>
<label for="female">
<input type="radio" id="female" name="gender" value="female"> Female
</label>
<label for="other">
<input type="radio" id="other" name="gender" value="other"> Other
</label>
</div>
</div>
<!-- Country Selection (Dropdown) -->
<div class="form-group">
<label for="country">Country</label>
<select id="country" name="country" required>
<option value="">Select your country</option>
<option value="India">India</option>
<option value="USA">USA</option>
<option value="UK">UK</option>
<option value="Canada">Canada</option>
<option value="Australia">Australia</option>
</select>
</div>
<!-- Terms and Conditions Checkbox -->
<div class="form-group">
<label>
<input type="checkbox" name="terms" class="check-agree" required> I agree to the <a href="#">terms and conditions</a>
</label>
</div>
<!-- Submit Button -->
<button type="submit" class="submit-btn">Sign Up</button>
</form>
</div>
</body>
</html>
Make sure to include the following input fields in your form:
- Text field for the full name
- Email input for the user’s email address
- Password input
- A submit button to send the form
This structure forms the foundation of the signup form. Once the structure is ready, it’s time to move on to the styling part with CSS code.
Step 2: Designing the Signup Form with CSS Code
After setting up the basic HTML structure, we will use CSS code to style and enhance the appearance of the form. CSS allows you to add visual elements like color, margins, padding, fonts, and responsiveness. This will make your signup form visually appealing and user-friendly.
COPY THIS CODE SNIPPET:
/* CSS code snippet */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
font-family: Arial, sans-serif;
background-color: #f4f4f9;
display: flex;
justify-content: center;
align-items: center;
padding:50px 0px;
}
.signup-container {
background-color: #fff;
padding: 25px;
border-radius: 10px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
max-width: 600px;
width: 100%;
}
.signup-form h2 {
text-align: center;
margin-bottom: 20px;
color: #333;
}
.form-group {
margin-bottom: 15px;
}
.form-group label {
display: block;
margin-bottom: 5px;
color: #333;
font-weight: bold;
}
.form-group input,
.form-group select {
width: 100%;
padding: 10px;
border: 1px solid #ccc;
border-radius: 4px;
font-size: 16px;
}
.gender-options {
display: flex;
justify-content: space-between;
margin-top: 5px;
}
.gender-options label {
display: flex;
align-items: center;
}
.gender-options input {
margin-right: 5px;
}
.check-agree{
width: fit-content !important;
}
.submit-btn {
width: 100%;
padding: 12px;
background-color: #28a745;
color: white;
border: none;
border-radius: 4px;
cursor: pointer;
font-size: 18px;
transition: background-color 0.3s;
}
.submit-btn:hover {
background-color: #218838;
}
.form-group a {
color: #007bff;
text-decoration: none;
}
.form-group a:hover {
text-decoration: underline;
}
/* Responsive Design */
@media (max-width: 480px) {
.signup-container {
padding: 20px;
width: 95%;
}
.form-group input,
.form-group select {
font-size: 14px;
}
.submit-btn {
font-size: 16px;
}
}
By adding CSS, you can:
- Centre the form on the page using Flexbox or Grid.
- Style the input fields to look clean and modern.
- Adjust the size and appearance of the submit button.
- Add hover effects to make the form interactive.
If you want your signup form to look professional, it’s essential to ensure good spacing, alignment, and consistent fonts. This makes your form easier to use and more visually attractive.
Step 3: Aligning the Form Using HTML and CSS Code
A crucial part of any form is its alignment. For users to fill out the signup form easily, all elements must be properly aligned. Using CSS Flexbox or CSS Grid can help ensure the form’s fields are aligned well.
Here’s how you can use HTML CSS code to align the signup form:
Table of Contents
/* Aligning the Form CSS Code */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
font-family: Arial, sans-serif;
background-color: #f4f4f9;
display: flex;
justify-content: center;
align-items: center;
padding: 50px 0;
}
.signup-container {
background-color: #fff;
padding: 25px;
border-radius: 10px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
max-width: 600px;
width: 100%;
}
.signup-form h2 {
text-align: center;
margin-bottom: 20px;
color: #333;
}
.form-group {
margin-bottom: 15px;
}
.form-group label {
display: block;
margin-bottom: 5px;
color: #333;
font-weight: bold;
}
.form-group input,
.form-group select {
width: 100%;
padding: 10px;
border: 1px solid #ccc;
border-radius: 4px;
font-size: 16px;
}
.gender-options {
display: flex;
justify-content: space-between;
margin-top: 5px;
}
.gender-options label {
display: flex;
align-items: center;
}
.gender-options input {
margin-right: 5px;
}
.check-agree {
width: fit-content !important;
}
Proper alignment makes a huge difference in terms of user experience. A well-aligned signup form will not only look better but will also be more functional.
Step 4: Making the Signup Form Responsive
In today’s world, most users access websites through their phones. It’s crucial that your signup form works well on mobile devices too. This is where CSS media queries come in handy.
By adding media queries in your CSS code, you can adjust the form’s layout and size based on the screen size. Here’s how you can make your form template in HTML CSS responsive:
- Use
@media
queries to detect screen size. - Adjust the width of the input fields for smaller screens.
- Stack the form fields vertically when viewed on mobile devices.
You can place your responsive CSS code snippet here:
/* Responsive Design */
@media (max-width: 480px) {
.signup-container {
padding: 20px;
width: 95%;
}
.form-group input,
.form-group select {
font-size: 14px;
}
.submit-btn {
font-size: 16px;
}
}
With this step, your signup form will look good on all devices, from large desktops to small smartphones.
Step 5: Finalizing the Signup Form Template in HTML CSS
Once you’ve structured, designed, aligned, and made the form responsive, your signup form is almost complete! Now, you can finalize your form template in HTML CSS by adding finishing touches like:
- Proper error handling using HTML attributes (e.g.,
required
). - Placeholder text in input fields to guide users.
- Customizing the submit button for a call-to-action (e.g., “Sign Up”).
With this, your form is ready for use. Now users can easily fill out and submit the form, and it will look clean and professional on all devices.
Best Practices for Creating a Signup Form
Now that you’ve learned how to create a signup form using HTML CSS code, here are some best practices to keep in mind:
- Use Clear Labels: Always provide clear labels for input fields to make it easier for users to understand what information is required.
- Keep It Simple: Don’t overload the form with too many fields. Keep it simple and ask for only the essential information.
- Ensure Accessibility: Use accessible attributes like
aria-label
to make sure the form is usable by all, including people with disabilities. - Add Form Validation: Use HTML form validation (e.g.,
required
,email
) to ensure users provide the correct data before submission. - Design for All Devices: Always make sure your form is responsive and looks good on both desktops and mobile devices.
Conclusion
Building a signup form using HTML and CSS is an essential skill for any web developer or student learning web development. By following the steps outlined in this guide, you now know how to create a functional and well-designed form using HTML CSS code.
Whether you’re creating a basic form template in HTML CSS or a more complex registration system, the key is to focus on structure, design, and responsiveness. With these skills, you can create signup forms that are both visually appealing and user-friendly.
Now that you know how to create a signup form using HTML and CSS, it’s time to get creative! Feel free to add additional fields, custom designs, or even JavaScript functionality to further enhance your form. Happy coding!
login page with an option to switch to the signup form. When you click “Sign up,” the signup page will appear, and clicking “Login” will toggle back to the login
[…] the projects listed here are beginner-friendly and include ideas that help you learn and apply new technologies […]